Yes, it is very pretentious title, but throughout my career, that could be considered as in mid-tier length, there are always some kind of topic that all the industry tries to focus on. Maybe that is selective perception of me but I think a lot of people thinks in this way. Microservices, machine learning, artificial intelligence, big data, virtual reality, blockchain. The list of tech buzzwords can be extended and the last one that I came across recently is “Reactive programming”.
I will examine reactive programming in satisfying detail both technically and practically, because I am going to make a presentation in my company. As a lazy person, if there’s an opportunity to kill two birds with one stone, I’d never miss it. So this is why I am writing about reactive programming today.
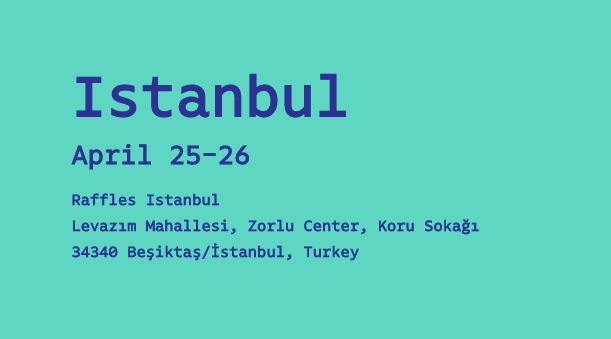
My reactive interest started with the conference held in Istanbul by Pivotal, the team behind famous Spring framework. The conference was two day long and mostly about Reactive Programming along with the activities done by company. Since the Pivotal is a cloud services company, half of the talks are about DevOps tools and how to effectively use them, but Reactive part of the talks really catch my attention.
I will not talk too much about conference, but I would like to draw attention to some part of it. Josh Long and Stephan Nicoll who are the two of the major developer had part in developing Spring framework, gave a talk almost half a day long about Reactive part of the Spring and the effect of Reactive Programming in industry. This event gave me the perception that I could hear the name of Reactive programming in many places in a short period of time.
What is Reactive Programming?
Although Reactive Programming is not new in industry, there are limited number of resources to understand. The available resources address reactive programming from different perspectives, and some are too technical, making it difficult to understand what it is. Stackoverflow approved answer is not suitable for newcomers, Reactive Manifesto is too formal and is a kind of explanation that for a project manager, Wikipedia( no link ಠ~ಠ ) is too shallow and theoretical and Microsoft Rx explanation is too complex.
To describe it in a very basic way, Reactive Programming is programming with async data streams. Actually if you code in javascript you are familiar with the Reactive Programming in a way. Below there is a piece of code that binds a click event to a DOM element, and whenever there is a click, attached function reacts to it by writing “Hello World!“. Also, Promises in async programming in javascript is very similar to reactive programming. We can think reactive programming is a promises with super powers.
1element.addEventListener("click", myFunction);23function myFunction() {4 alert ("Hello World!");5}
Let’s analyze this simple code in the aspect of RP. There is a observer which following specific events to be happen and reacts it, observable who is being followed for any action. When specific event happens, observable emits and warns all the observers who is previously subscribed to observable’s event. That was a lot of words. It can easily be understood by looking at below diagram. Simply, it is good old Observer Pattern.
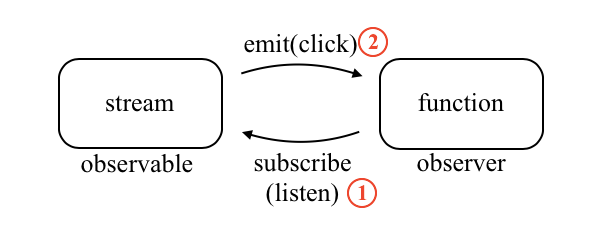
Before going deep into reactive programming I would to point out something. Remember that I said Reactive Programming is not new and also I said that there is not enough resources since it is relatively new. Did I contradict with myself? Actually, no. When people often say reactive programming, they actually mean Functional Reactive Programming(FRP), which is combination of reactive programming (everything is data stream) and functional programming (everything is pure function). Best of the both world, sweet!
Streams
In order to understand Reactive Programming, we have to look streams in a more detailed way. As I said before, RP is all about streams. Stream is a sequence of an objects and accessed in sequential order. Simply reading a file from file system is an example for streams. We read it byte by byte until end of file symbol. Streams are introduced in Java 8 for JDK. There are some functional methods for Streams in order to interact with them. Usually these operations take a stream as input (source), make some operations (intermediate operation) which transforms into a new stream and finally returns a final result (terminal operation). This is called stream pipeline.
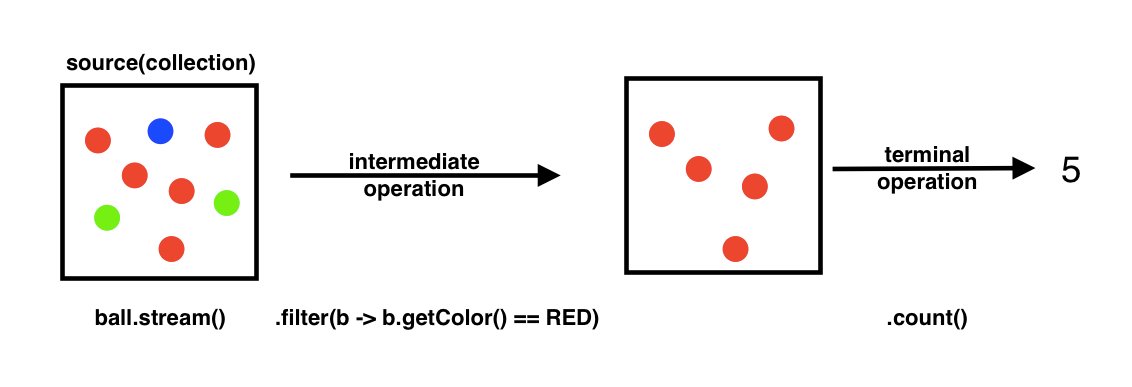
Passive Programming vs Reactive Programming?
Comparing things and giving concrete examples makes it easier to understand. So, let’s analyse reactive programming with an e-commerce site. Below there is a diagram for Cart and Invoice, when a new product added to cart, number of items in invoice and total amount should be updated accordingly.
In the first example, updating invoice responsibility given to Cart class. Whenever a new item added, it updates invoice and Invoice class does nothing actively. In the second example Invoice listens for Cart public event changes and updates itself when one occurs. In the passive programming there are remote setters and updates while in reactive programming there is event observation and self-updates.
Imagine a bigger system full of classes and methods. In passive programming in order to find a usage of class and its functionality someone has to look other classes which uses that functionality. For instance at the first diagram below, in order to find Invoice usage programmer needs to look at Cart and Coupon classes. Meanwhile, in reactive programming in order to find class usage and functionality simply someone should look inside the class. In the second diagram it can be observed, for Invoice class functionality it is enough to look inside it. This situation immediately reminds me Tell, Don’t Ask (Information Expert) principle originally introduced by Craig Larman in his famous book: Applying UML and Patterns and explained by Martin Fowler in his article. In Tell Don’t Ask principle it is suggested that all data and methods/functionalities should be encapsulated in a single object. Even though passive programming is a better tool for dependency injection and data structures, providing this principle out of the box made reactive programming more adaptive for Object Oriented Design.
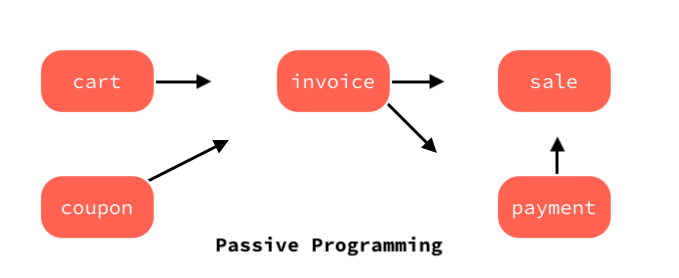
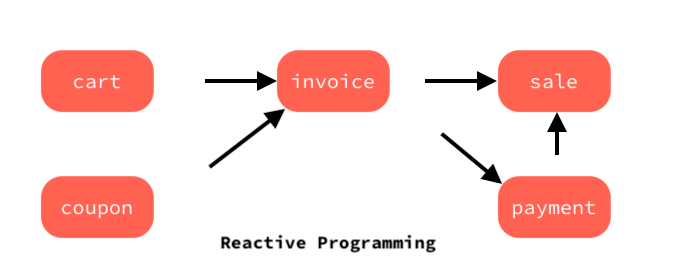
Also, having this kind of isolated set of functionalities could make it easier to implement aspect oriented programming with reactive approach. For instance, all analytics related operation could be encapsulate in a component, and this component observes all the point cuts in the application.
Why Reactive Programming?
Twenty years ago, a website consists of a simple form in the aspect of functionality and considering the number of visitors, capacity of servers were not pushed to its boundaries. However, today even a simple website should contains a lot of functionalities along with auxiliary ones like caching, authentication, geolocation, cookies, analytics and still has to offer adequate performance. This is where reactive strategy comes into play. It solves problem like handling more users with the same resources, handling more inputs from user and making system easy to scale. How it can achieve this, let’s analyze with concrete example of users sending request to server.
Here is a simple Spring boot controller that has a repository with any type of database related and a simple request mapping that brings user with given id.
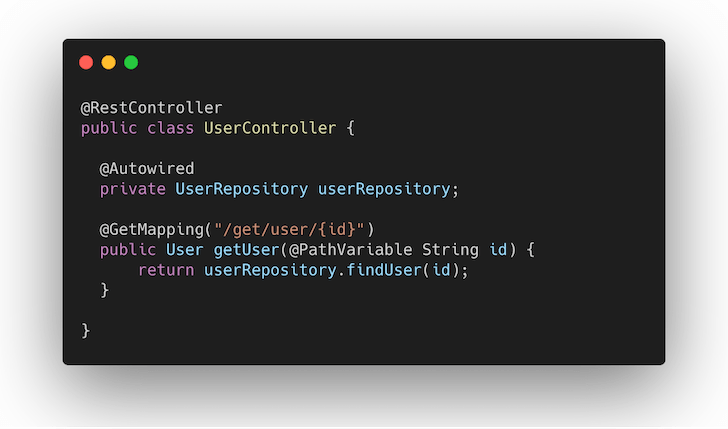
In traditional way when a request came to the server first it has assigned to a servlet thread. This thread make some processing on this request and then redirects to database driver. While database operation is executing this particular thread waits database to finish operation. During this operation thread could not be able to detach from connected request and blocked until database response. In JVM there is a pool in which all threads are kept and there is a limit for this pool. Let’s there can be simultaneous 200 different thread at the same time. When 200 request came from client, the request after that has to wait for database operation to terminate. This reduces scalability for system and the number of available threads at the same time creates a upper bound.
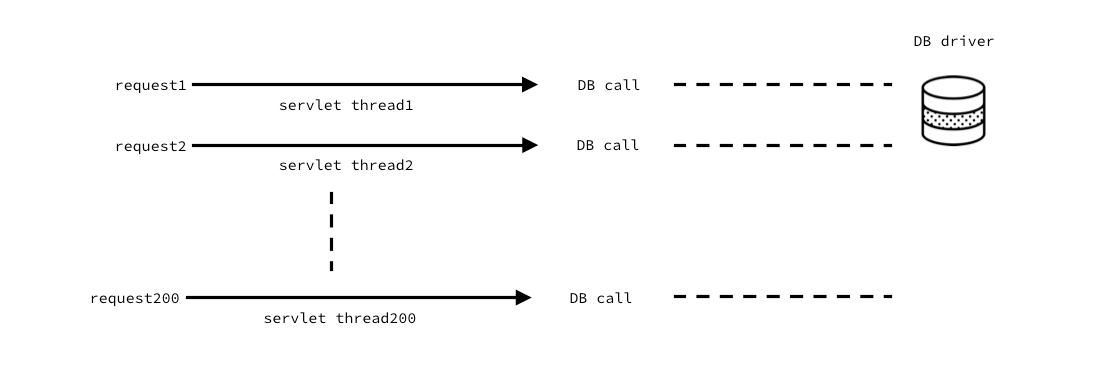
When we encounter this problem in reactive programming, it can be solved by using streams and non blocking database drivers. In our passive Spring controller there was a blocking database driver used in UserRepository
. Spring introduces a new framework for spring boot applications called WebFlux along with non blocking database drivers for databases like mongodb, cassandra and redis. By changing database driver in UserRepository
and return type of getUser method to Mono<User>
.
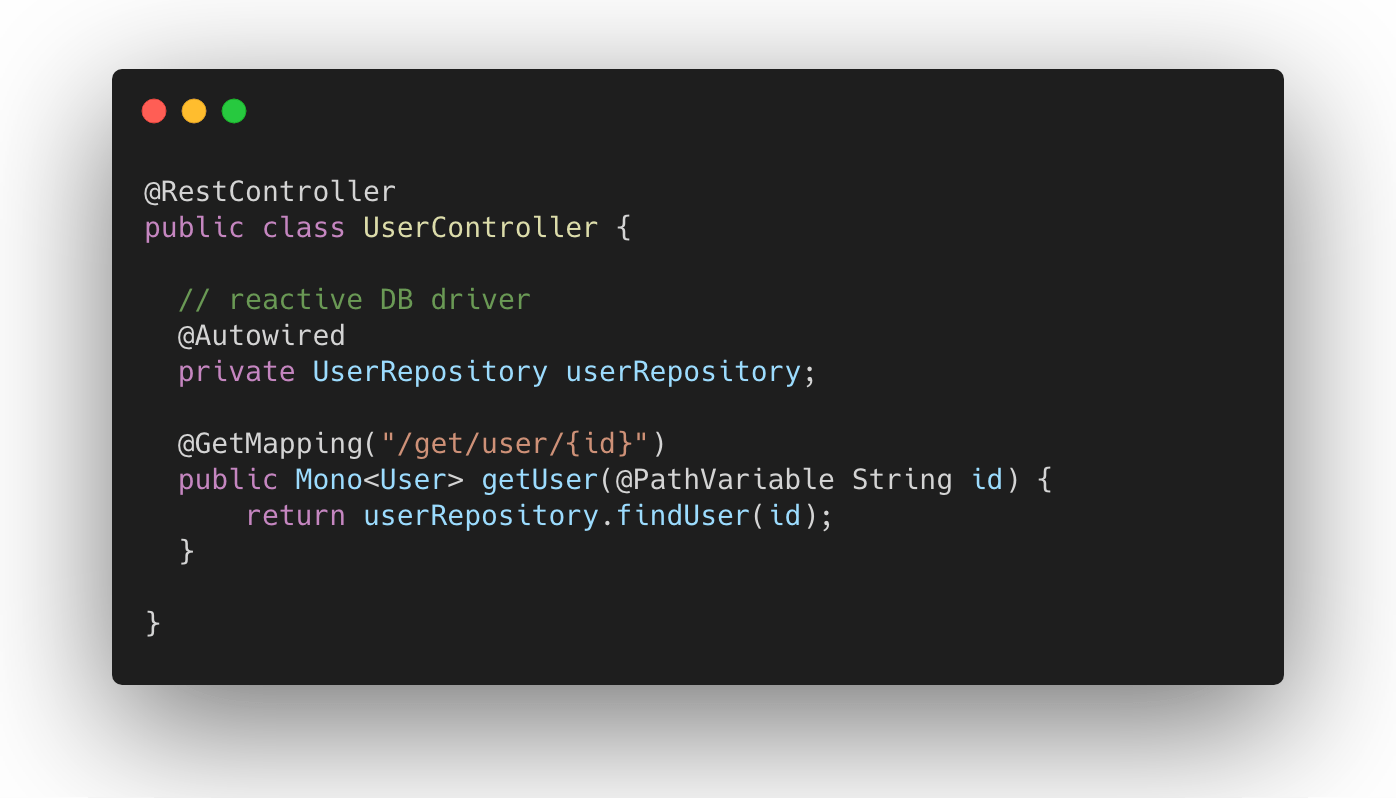
With the updated code and reactive strategy, now we can serve more users and handle more inputs with same amount of resources or even less. Whenever there is a new request sent to the server as in the previous example, firstly it is attached to servlet thread. However with non blocking database driver, now the thread calling the database operation does not have to wait for process to complete. It can bring another request to the database to execute or if the first request is executed and ready to return to client, it could return it.
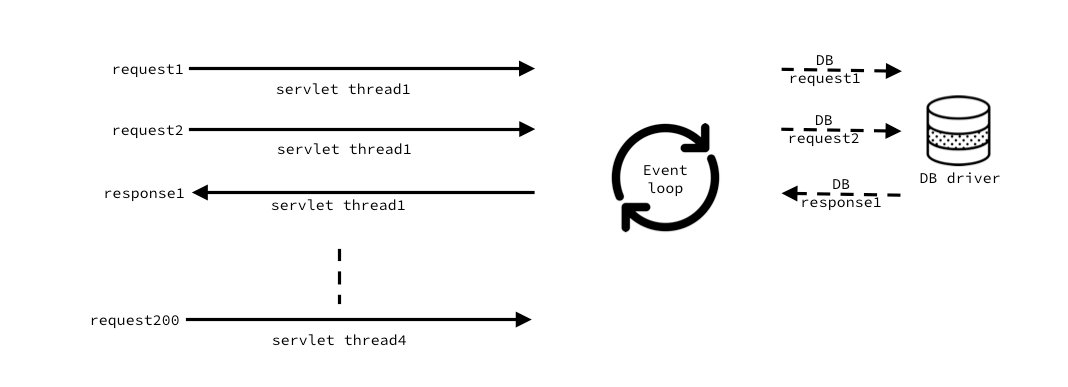
Even looking at this improvement, reactive programming can increase scalability in a great way. However when we investigate a little more, it can be seen there are more performance improvement. Threads are very expensive for an operation system in the aspect of required time to switch between contexts. There are a lot of operation during switching like saving current context, recalling previous context of new thread, determining which operation to execute and required memory operations. By using reactive strategy this overhead can be decreased dramatically since it does not require a lot of context switching.
Reactive in Java
There are two famous framework for reactive programming in java: RxJava and Spring WebFlux. Both of the framework depends on reactive streams written for JVM. RxJava is the first extension for Java and works with Java 6+, provides asynchronous event based programming in Java and Android Java. Spring WebFlux is more popular since it is works with Java 8+ and the built in support for Spring framework.
There are four fundamental things to learn in Spring WebFlux: Flux and Mono, Publisher and Subscriber. Flux is the stream of 0 to N elements and Mono 0 or 1 element. At first, there was only Flux but later it is understood that, there is a need for a stream that only consist of 1 element. Both Mono and Flux is an implementation of Publisher class. So, in order to data to be flow, they have to be subscribed by other subscribers. Let’s put these words into code example.
1Mono<String> mono = Mono.just("foo");2Flux<String> flux = Flux.just("foo", "bar", "baz", "qux");34// interface in place of Mono and flux5Publisher<String> m = Mono.just("foo");
In order to understand easily Publisher and Subscriber, assume there is an User
entity and a userMongoRepository
which is a Reactive MongoDB implementation and there is a stream of consisting 4 User
. Each user is saved to non-blocking database. Since save
methods’ return type is Mono, which is a Publisher, it can be subscribed. After completing save operation, as a Publisher it emits signal and subscriber reacts to that signal, in this case subscriber is print operation. This whole process is a very basic example of Reactive Programming in Spring WebFlux.
PS: System.out::println
is method reference of lambda operation: str -> System.out.println(str)
1Stream.of(2 new User(UUID.randomUUID().toString(), "A"),3 new User(UUID.randomUUID().toString(), "B"),4 new User(UUID.randomUUID().toString(), "C"),5 new User(UUID.randomUUID().toString(), "D"))6 .forEach(user -> userMongoRepository.save(user)7 .subscribe(System.out::println)));